What is the difference between var, let and const in JavaScript?
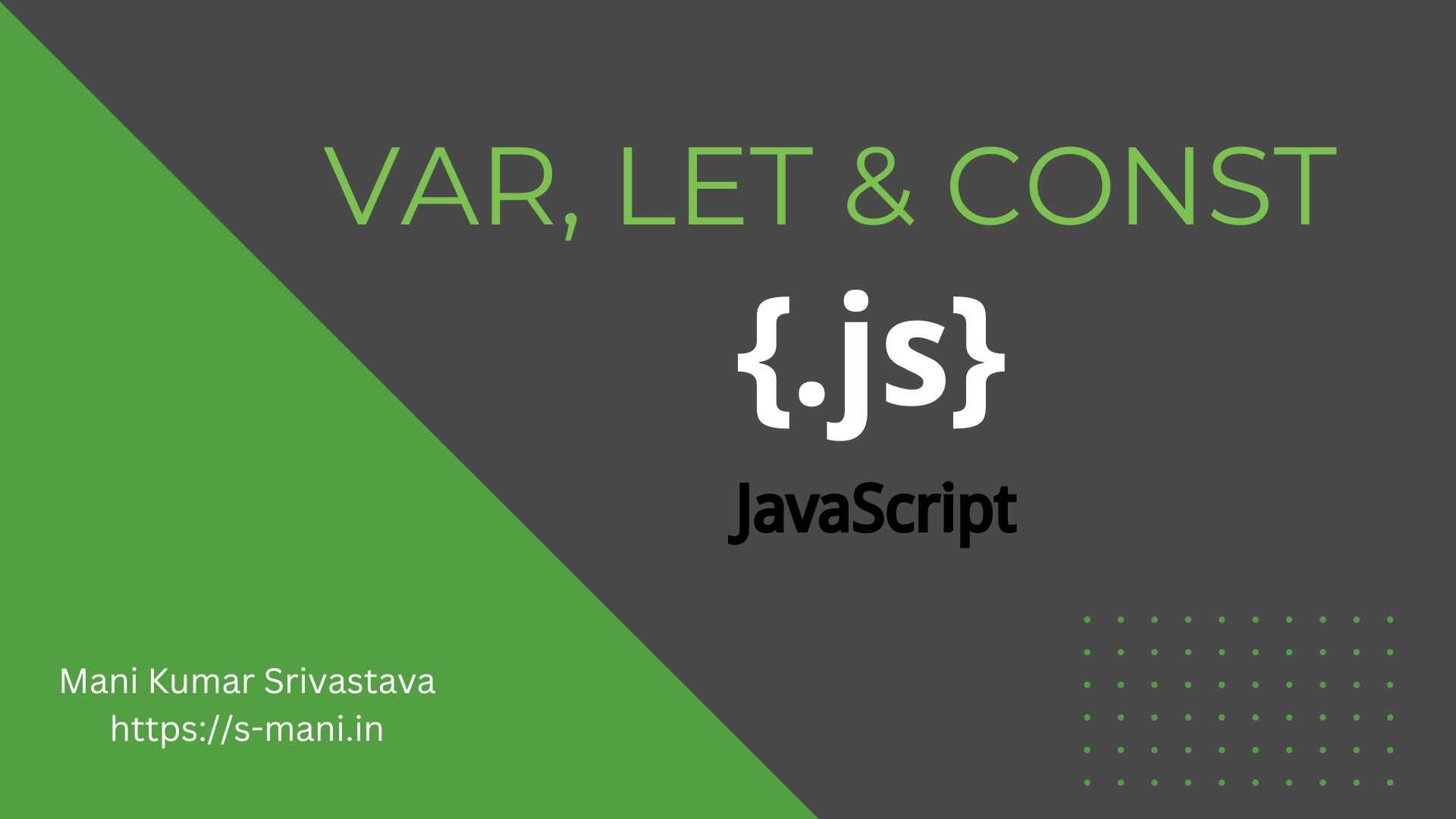
var, let and const are ways to declare a variable in JavaScript. var has been there since the beginning of JS, and other two were introduced in ES2015 to overcome the shortcomings of it.
This article compares the three on following factors -
- Variable Scope
- Redeclaration and Reassignment
- Hoisting
Variable Scope
In programming, scope refers to the part/subset of code within which a variable or a function is available to be accessed.
There are four types of scopes -
- block scope
- local scope (or function scope)
- script scope
- global scope
Note
There are few more types of scope, such as closure scope, but those are out of the "scope" of this article.
Note
During execution of the code, you can check what is the scope of a variable in the Chrome Dev Tools.
Follow these steps -
- Open the dev tools. Do right click and then select "Inspect"
- Go to the "Sources" tab
- In the right panel, there must be a sub-panel with the title "Scope"
You have to stop the execution of the code at that specific point where you want to check the scope of a variable. You can use "debugger".
#1 Block Scope
Code that is written between curly braces ({..}), except functions, is called as a CODE BLOCK
Following are the examples of code blocks -
All these are called Code Blocks.
A variable is said to have the Block Scope, when a variable declared within the code block can be accessed only inside the code block, not outside of it.
#2 Local Scope (or Function Scope)
A variable is said to have Local scope if it is declared inside a function. That variable can not be accessed outside of it.
#3 Script Scope
A variable is said to have script scope if it is declared outside of any block or any function. In other words, the variable should be declared at the top level of the js file. For example -
This implies that that variable can be accessed by any code block and any function in that file.
Note
One interesting thing to note is that a variable having script scope can be accessed by other js files also, given that file declaring that variable should come before the file, which accesses it, in HTML.
#4 Global Scope
A variable is said to have global scope if it is declared outside of any block or any function. It is almost same as script scope, that it can be accessed inside block, function and even in other js files, but has one small difference. Global scope variables become a part of window object.
Note
All these definition of scope will make sense with the help of examples, which are mentioned below.
Note
Variables declared with -
- var : can have local and global scope.
- let & const : can have block, local and script scope.
Let's understand with the help of examples
Note
Examples shown with "let" are also applicable for "const"
Redeclaration and Reassignment
Declaration Type | Description |
---|---|
var | Variables declared with var can be redeclared and reassigned in its current scope. |
let | Variables declared with let can be redeclared in other scope than the current one. But it can be reassigned in current and any child scope. |
const | Variables declared with const can neither be redeclared nor reassigned any where. |
Note
If you don't declare a variable with either var, let or const, then automatically JS assumes it is var type variable, and it becomes the part of global scole, even though that piece of code lies in the function.
Let's look at few examples
Hoisting
According to MDN documentation,
JavaScript Hoisting refers to the process whereby the interpreter appears to move the declaration of functions, variables, classes, or imports to the top of their scope, prior to execution of the code.
Variables declared with var are hoisted to the top of the global and local scope.
Please look at the following code and its output
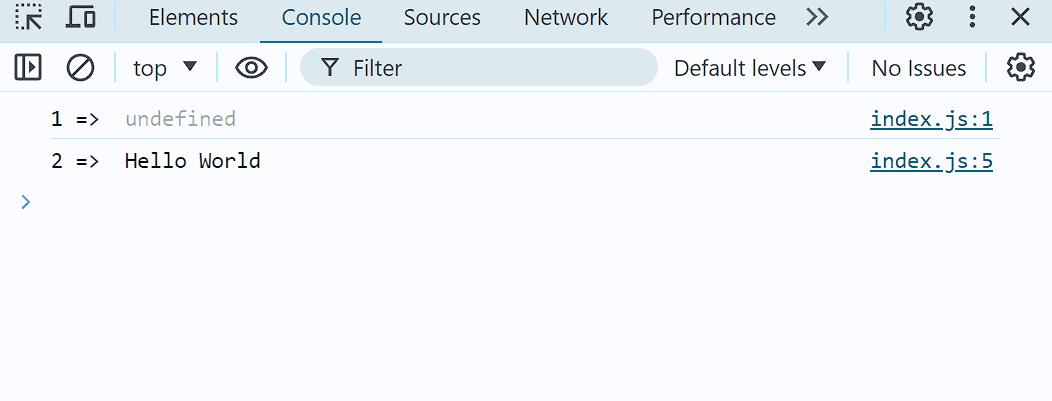
It implies that var variables are moved up to their scope and are assigned with undefined. And since we know that var variables can be reassigned, it takes up the value later on.
Variables declared with let and const, technically, also hoist up to their scope. But they throw the error if they are accessed before they are declared.
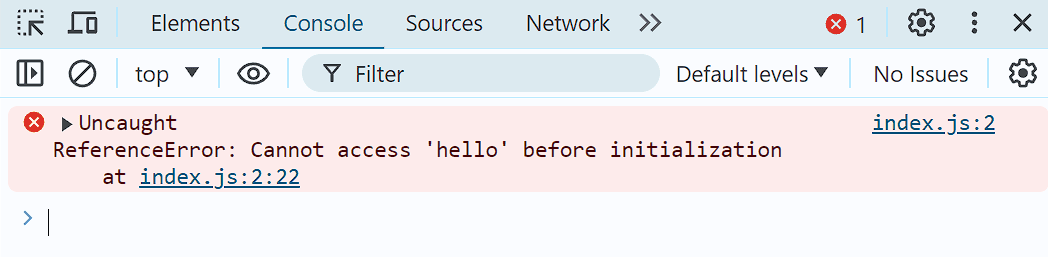
The results are same for variable declared with const.
Conclusion
In this article, we saw the differentiating factors of var, let and const in JavaScript.
One extra important thing to note is that JavaScript sequentially searches for a variable from its current scope to higher scopes until the value is found.
Note
The order of scope is as follow -
If a variable is not found in one scope, then it looks for the variable in the next scope, and so on, until the value is found. If it is not, then it returns back undefined.